Spring Bean Life Cycle
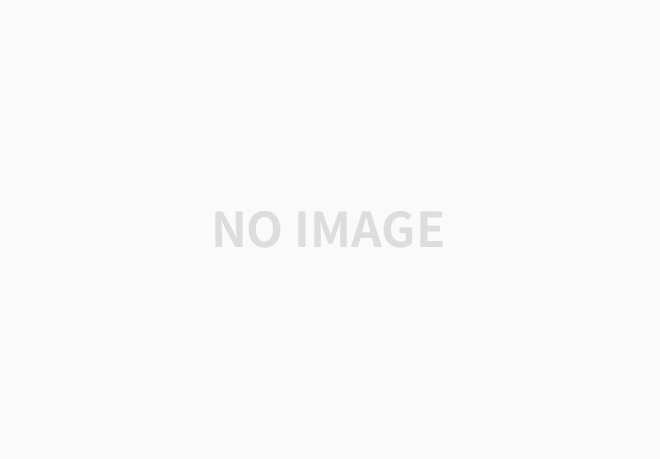
Annotation을 이용하는 방법
@PostConstruct, @PreDestroy 를 이용하면 Bean이 생성될때 Bean 제거 될때의 Life Cycle에 코드를 실행을 할수가 있다.
@Service
public class MyService {
@PostConstruct
public void init(){
System.out.println("MyService init method called");
}
@PreDestroy
public void destory(){
System.out.println("MyService destroy method called");
}
}
InitializingBean, DisposableBean Interface를 이용하는 방법
Interface를 구현함으로써 빈이 생성이 될때 소멸이 될때에 코드를 실행할수가 있다.
@Service
public class MyService implements InitializingBean, DisposableBean
{
@Override
public void afterPropertiesSet() throws Exception
{
System.out.println("MyService init method called");
}
@Override
public void destroy() throws Exception
{
System.out.println("MyService destroy method called");
}
}
(2018. 12. 10 추가) Lifecycle Interface
public interface Lifecycle {
void start();
void stop();
boolean isRunning();
}
(2018. 12. 10 추가) SmartLifecycle Interface를 이용하는 방법
- 시작과 종료가 중요할때 사용되는 SmartLifeCycle, getPhase()를 정의함으로써 객체가 언제 start되는지 언제 stop이 되는지에 대한 순서를 정할수가 있다.
- 값이 클수록 나중에 start되고, 먼저 stop이 된다.
- stop 할때 runnable인 callback을 반드시 실행해줘야 비동기적으로 비동기 종료가 가능하다.
public class MyService implements SmartLifecycle {
private boolean running;
@Override
public void start() {
System.out.println("start");
running = true;
}
@Override
public void stop() {
running = false;
}
@Override
public boolean isRunning() {
return running;
}
@Override
public int getPhase() {
return 0;
}
@Override
public boolean isAutoStartup() {
return true;
}
@Override
public void stop(Runnable callback) {
stop();
callback.run();
}
}
'Programing > Spring Framework' 카테고리의 다른 글
Spring Retry (0) | 2018.10.30 |
---|---|
Spring 자체 인스턴스화 된 객체에 종속성을 주입하는 방법 (0) | 2018.10.20 |
Spring @Transactional Propagation (0) | 2018.09.10 |
Spring Constructor Dependency Injection (0) | 2018.09.06 |
Spring JDBC (0) | 2018.08.23 |